Don't blame the user
2015-07-21We (developers) are always working on avoiding our applications to break, crash or have any kind of bugs. But so far no one seems to have found a cure for these problems that our users face. But I believe that there are ways to reduce the pain four our users when they find the holes in our applications. And we should definitely not blame them when they get into problems because the application breaks down.
Let me give you an example of an application that blame the user, when it’s actually the application that broke down.
When I tried to login to a web application I got this error message:
Internal Server Error The server encountered an internal error or misconfiguration and was unable to complete your request. Please contact the server administrator, 3014@server_owner.com and inform them of the time the error occurred, and anything you might have done that may have cause the error. More information about this error may be available in the server error log.
The worst thing about this error message is that it’s trying to blame the user for error that happened. You see the blame in this part of the error message: “anything that you might have done that may have caused the error”. This is definitely making the customer / user feel that the problem that happened is his / her fault and feel sad and blame themselves for the problem. In this case it was definitely not the users fault; I only entered my username and password.
The second problem with this error message is that the company wants the user to contact them to tell them about the error that happened. This is very strange since this is a web application owned by the company and they put out the error message and that means that they should be able to log this problem them self.
This was just an example of a not so nice error message could look like, but there are loads of bad error messages out there in most applications.
How does the user feel after getting this error message? Probably a bit stupid and probably don't know he/she did wrong. I'm also pretty sure that the user will not help the website with any information, since the website tried to blame the user. This will hurt the feelings that the user has for the website and the brand that it is representing.
Better UX for application problems
So what can we as developers do to create a better user experience for our user when something goes wrong with our applications?
I actually have a simple rule that is general to all applications that users are interacting with.
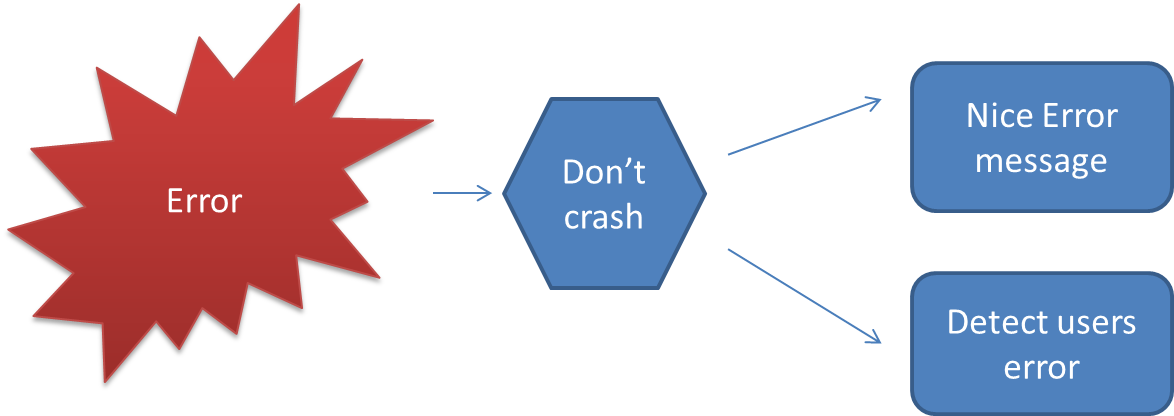
It always start with that the software encounters some kind of error, it could be everything from that the user entered an invalid value to a low level exception. As I said earlier there will always be unhandled or unexpected problems in an application, so you should always have ways in your application to handle these unexpected things in as nice way as possible for the user.
Don't crash
To begin with you need to try to avoid crashing the application in all possible ways. This is the most important rule of them all since if the application crashes you don’t have any way to notify or help the user in any way. So if the application crashes the user will be very surprised, annoyed and not knowing what happened. People really feel uncomfortable when they don’t know what happened. So telling the user that you had a problem and need to close the application is much better than just disappearing.
Techniques for staying alive when unexpected exceptions happen. These techniques are sometimes different depending on what application you have. Here I’m going to give two examples depending on if you are some kind of desktop application or if it’s a web application.
Application
Errors in applications can happen at many unexpected places. So putting a try catch block as high up as possible in your applications call stack is one of the best ways to avoid your application to crash. What do you actually do catch errors as high up as possible in your code?
You put a try catch block directly in the beginning in of your main method.
public static void Main(string[] args)
{
try
{
DoApplicationStuff();
}
catch (Exception e)
{
GiveUserNiceErrorMessage(e);
}
}
Example code done in C#.
Note: I really don’t mean that the only place in your code that you should put try catch blocks is in the main method. You should put them everywhere in your code where it’s necessary also.
Web
One thing that could happen on a website or web application is that the user goes to a URL that does not exist. Then the user could come to a default error page with the error code 404 "Page not found". To the end user this page probably looks like the web application crashed and user getts no direction on what todo or how to get out of this dead end.
It's possible to create a custom 404 page, so that you as the web application owner can give your users a better experience when a page link is broken and give them a good error message.
If you are using the IIS webserver, this is what you need do in the web.config file to enable a custom 404-page.
In the section <system.web> this markup needs to be added:
<customErrors mode="On">
<error statusCode="404" redirect="/404-error.aspx"/>
</customErrors>
Then under the section <system.webServer> this markup needs to be added:
<httpErrors errorMode="Custom">
<clear/>
<error statusCode="404" path="/404-error.aspx" responseMode="Redirect"/>
</httpErrors>
What you have done now with this configuration change is to disable the default 404-page and instead redirect a 404-error to the 404-error.aspx page. This means that you now need to create a file called 404-error.aspx where you can add your custom error message to help your users.
Great now you have the basic level of safety net, so you will be able to sow your user an error message. The next section will guide you in how to write good error messages.
Nice error messages
You could categories exceptions into two types, one where you know what the exception is and you know how to tell the user how to handle it and the other one is when you have not written any specific code to handle the exception, so don’t know what to tell the user.
Known Exceptions
A simple example of a known exception is if a user puts SWEDEN in the field for the phone number. Then you can easily check of the field only contains numbers and if it does not you need to inform the user about it.
A good practice is then to give the user an error message that answers these two questions for the user:
- What is wrong?
- How can the user fix what is wrong?
Example of a good error message:
You entered a Year of Birth that is in the future. You can fix this by entering your Year of Birth and that should be in the passed.
Unknown Exceptions
This is a more interesting type of exception and it’s also most likely a bug in the in the application that needs to be fixed. An unknown exception is a exception that you have not written any specific code to handle.
So what can we tell the user when a unknown exception is raced? Should you give the user a error code and tell them that they did something wrong and that they should contact your customer support to report the problem? The answer to this is no if you want o have happy user.
Good practice for unknown exceptions:
- Rule number one, be nice to the user and apologize for the problem in the application.
- Guide the user on what to do next
- Send the exception back to you so that you can start to look at the problem (tell also the user about this)
Don't forget to test out error message with user to see that they understand it and know what to do when they get it. Error message are also something that needs testing and continues improvement.
How should the error message have been written? Let’s take the take login error that I got, that got me started to write this blog post as an example. So that Internal Error message could have been written maybe like this:
Ops, looks like we have some problem with login What you can do now: - Try again in a little while to see if we have been able to fix the problem. - Continue to use the site with the content that does not require a login. This problem has been sent to our support department that will investigate the problem and fix it.
A Classic example of 404 error message from GitHub that has some humor to it also. It's not a perfect example on how to write this kind of message but it's a bit funny.
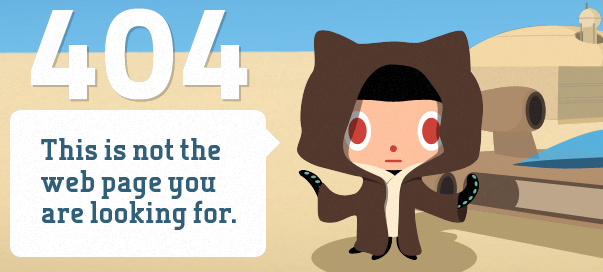
Detect user errors
To be one step ahead you should always try to detect when a user experience an exception or an error. The ultimate goal for you is that you should already be working on the problem that your user experienced before they call you or write something nasty about the error on Twitter. If you are already working with the problem you can very quickly give a response to what the problem is about and hopefully also when you can fix it. If you manage to give such an answer, the user will feel that you have the situation under control and much more confident that they did not blame them self for causing this error.
There are many ways to capture and send exceptions back to, there are also a lot of tools that can help you with this. But I will not bring those up in this blog post.
Keep the user happy
To summarize what I have said in this post you should think of these things to keep your user happy. It's not possible to avoid having bugs in your applications, so you need to be nice to users when they hit a exception. When they get an exception you should take the blame for the problem and try to offer them a solution on how to continue from there. Also send back the information about the exception back to you, so that you can fix the problem for the user without them having to report the problem to you.
With these advices you can continue to have happy user even if they find your bugs!
Interesting Links: